topical media & game development
Stacks and stacks of books on DirectX
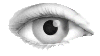
1
DirectX 9 SDK
Many of the multimedia applications that you run on your PC,
to play games, watch video, or browse through your photos,
require some version of Direct X to be installed.
The most widely distributed version of Direct X is 7.0.
The latest version is the october release of 2004.
This is version 9c.
What is DirectX?
And, more importantly, what can you do with it?
In the DirectX documentation that comes with the SDK, we may read:
DirectX
Microsoft DirectX is an advanced suite of multimedia application programming interfaces
(APIs) built into Microsoft Windows; operating systems.
DirectX provides a standard development platform for Windows-based
PCs by enabling software developers to access specialized hardware features without
having to write hardware-specific code.
This technology was first introduced in 1995 and is a recognized standard
for multimedia application development on the Windows platform.
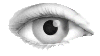
Even if you don't use the DirectX SDK yourself, and to do that you must be
a quite versatile programmer, then you will find that the tools or plugins that
you use do depend on it.
For example, the
WildTangent game engine plugin makes the DirectX 7 functionality available
through both javascript and a Java interface.
So understanding what DirectX has to offer may help you in understanding and exploiting
the functionality of your favorite tool(s) and plugin(s).
DirectX 9.0 components
In contrast to OpenGL, the DirectX SDK is not only about (3D) graphics.
In effect, it offers a wide range of software APIs and tools to
assist the developer of multimedia applications.
The components of the DirectX 9 SDK include:
DirectX 9 components
- Direct3D -- for graphics, both 2D and 3D
- DirectInput -- supporting a variety of input devices
- DirectPlay -- for multiplayer networked games
- DirectSound -- for high performance audio
- DirectMusic -- to manipulate (non-linear) musical tracks
- DirectShow -- for capture and playback of multimedia (video) streams
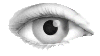
In addition there is an API for setting up these components.
Also, DirectX supports so-called
media objects, which provide
a standard interface to write audio and video encoders, decoders and effects.
Altogether, this is a truly impressive and complex collection of APIs.
One way to become familiar with what the DirectX 9 SDK has to offer
is to start up the sample browser that is part of the SDK and explore the demos.
Another way is to read the online documentation that comes with the SDK,
but perhaps a better way to learn is to make your choice from the large
collection of introductory books, and start programming.
At the end of this chapter, I will provide some hints about how to get on your way.
Direct3D
In the DirectX 9 SDK, Direct3D replaces the DirectDraw component of previous versions,
providing a single API for all graphics programming.
For Direct3D there is a set of simple, well-written tutorials in the online documentation,
that you should start with to become familiar with the basics of
graphics programming in DirectX.
Direct3D tutorials
- tutorial 1: creating a device
- tutorial 2: rendering vertices
- tutorial 3: using matrices
- tutorial 4: creating and using lights
- tutorial 5: using texture maps
- tutorial 6: using meshes
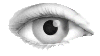
Before you start working with the tutorial examples though,
you should acquire sufficient skill in C++ programming
The DirectX 9 SDK also offers APIs for C# and VisualBasic .NET.
See the research directions at the end of this section.
and also some familiarity with Microsoft Visual Studio .NET.
One of the most intricate (that means difficult) aspects of
programming Direct3D, and not only for novices,
is the creation and manipulation of what is called the device.
It is advisable to take over the default settings from an example,
and only start experimenting with more advanced setting
after you gained some experience.
DirectSound -- the drumpad example
The DirectX SDK includes various utility libraries,
for example the D3DX library for Direct3D, to simplify DirectX programming.
As an example of a class that you may create with DirectSound, using
such a utility library, look at the drumpad below.
The drumpad class can be integrated in your 3D program,
using DirectInput, to create your own musical instrument.
The header of the class, which is, with some syntactical modifications, taken from
the SDK samples section, looks as follows:
class drumpad {
public:
drumpad()
~drumpad();
bool initialize( DWORD dwNumElements, HWND hwnd );
bool load( DWORD dwID, const TCHAR* tcszFilename );
bool play( DWORD dwID );
protected:
void CleanUp();
CSoundManager* m_lpSoundManager;
CSound ** m_lpSamples;
};
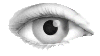
The interface offers some methods for creating and destroying a drumpad object,
initialization, loading sounds and for playing the sounds that you loaded.
The CSoundManager is a class offered by the utility library for DirectSound.
The play function is surprisingly simple.
bool drumpad::play( DWORD id ) {
m_lpSamples[id] -> Stop();
m_lpSamples[id] -> Reset();
m_lpSamples[id] -> Play( 0, 0 );
return true;
}
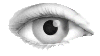
The id parameter is a number that may be associated with
for example a key on your keyboard or some other input device.
Using the drumpad class allows you to make your own VJ program,
as I did in the system I will describe in the next section.
In case you are not familiar with either C++ or object-oriented programming,
you should study object-oriented software development first. See for example [OO].
DirectShow
DirectShow is perhaps the most powerful component of the DirectX SDK.
It is the component which made Mark Pesce remark
that with the DirectX 9 SDK digital convergence has become a reality.
It is historically interesting to note that Mark Pesce may be regarded
as the inventor, or initiator, of VRML, which was introduced in 1992
as the technology to realize a 3D web, as interlinked collection of 3D spaces.
A technical reality, that is, [Video].
As we have seen in chapter 3,
working with multimedia presents some major challenges:
multimedia challenges
- volume -- multimedia streams contain large amounts of data, which must be processed very quickly.
- synchronization -- audio and video must be synchronized so that it starts and stops at the same time, and plays at the same rate.
- delivery -- data can come from many sources, including local files, computer networks, television broadcasts, and video cameras.
- formats -- data comes in a variety of formats, such as Audio-Video Interleaved (AVI), Advanced Streaming Format (ASF), Motion Picture Experts Group (MPEG), and Digital Video (DV).
- devices -- the programmer does not know in advance what hardware devices will be present on the end-user's system.
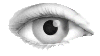
The DirectShow component was designed, as we learn from the online documentation, to
address these challenges and to simplify the task of creating applications by
isolating applications from the complexities of data transports, hardware differences
and synchronization.
The solution DirectShow provides is a modular architecture that allows
the developer to set up a data flow graph consisting of filters.
Such filters may be used for capturing data from, for example, a video camera or video file,
for deploying a codec, through the audio compression manager (ACM) or video compression manager (VCM) interfaces,
and for rendering, either to the file system or in the application
using DirectSound and DirectDraw and Direct3D.
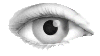
2
The diagram above,
taken from the DirectX 9 SDK online documentation,
shows the relations between an application,
the DirectShow components,
and some of the hardware and software components that DirectShow supports.
An interesting and convenient feature of the filter-based dataflow architecture of DirectShow
is SmartConnect, which allows the developer to combine
filters by indicating constraints on media properties such as format.
The actual connections then, which involves linking input pins to output pins, is done
automatically by searching for the right order of filters,
and possibly the introduction of auxiliary filters to make things match.
|
DepthCube, see example(s) -- 3D vision |
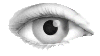
3
DirectX application development
The examples that come with the DirectX`9 SDK use an application utility library,
which includes a general application class that takes care of most of the details
of creating an application and rendering window, initialization and event handling.
For each of the SDK components there are numerous examples, ranging in difficulty
from beginners to expert level.
There are also a number of examples that illustrate how to mix
the functionality of different SDK components, as for example the projection of video on 3D,
which we will discuss in more detail in the next section.
|
|
|
3D vision | Perspectra | DepthCube |
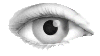
4
example(s) -- 3D vision
Have you ever wondered how it would feel to be
in Star Trek's holodeck, or experience your
game in a truly spatial way, instead of on a flat LCD-display.
In [DEEP], an overview is given of technology
that is being developed to enable
volumetric display of 3D data, in particular the
Perspecta swept-volume display (middle) and LightSpace DepthCube (right),
that uses a projector behind a stack of 20 liquid-crystal screens.
The first approach of displaying volumetric data,
taken by the Perspecta swept-volume display, is to project a sequence
of images on a rotating sheet of reflective material to
create the illusion of real volume.
The psychological mechanism that enables us to see
volumes in this way is the same as the mechanism that forces us
to see motion in frame-based animation, at 24 frames per second,
namely persistence of vision.
LightSpace DepthCube uses a stack of 20 transparent screens
and alternates between these screens in a rapid way,
thus creating the illusion of depth in a similar way.
In comparison with other approaches of creating depth illusion,
the solutions sketched above require no special eyewear
and do not impose any strain on the spectator due
to unnatural focussing as for example
with autostereoscopic displays.
For rendering 3D images on either the Perspecta
or DepthCube traditional rendering with for example OpenGL
suffices, where the z-coordinate is taken as an indication for
selecting a screen or depth position on the display.
Rendering with depth, however, comes at a price.
Where traditional rendering has to deal with,
say 1024x748 pixels,
the DepthCube for example needs to be able to
display 1024x748x20, that is 15.3 million, voxels
(the volumetric equivalent of a pixel)
at a comparable framerate.
research directions -- the next generation multimedia platform
Factors that may influence your choice of multimedia development platform include:
- platform-dependence -- both hardware and OS
- programming language -- C/C++, Java, .NET languages
- functionality -- graphics, streaming media
- deployment -- PC/PDA, local or networked, web deployment
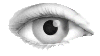
A first dividing line is whether you prefer to develop on/for Linux or Microsoft windows.
Another dividing line, indeed, is your choice of programming language, C/C++, Java or
.NET languages.
Another factor that may influence your choice is the functionality you strive for.
For example, Managed DirectX, for the .NET languages,
provides only limited support for DirectShow and does not allow for
capturing live video from a DV camera.
And finally, it matters what deployment you wish to target for,
mobile phone, PDAs or PCs, and whether you plan to make stand-alone applications or applications
that must run in a web browser.
Apart from the hard-core programming environments such as
the Microsoft DirectX 9 SDK, the Java Media Framework,
OpenGL with OpenML extensions for streaming media,
or the various open source (game development) toolkits,
there are also high-level tools/environments, such as Macromedia Director MX,
that allow you to create similar functionality with generally less effort,
but also less control.
In appendix E, a number of resources are listed that may assist you in determining your choice.
Given the range of possible options it is futile to speculate
on what the future will offer.
Nevertheless, whatever your choice is, it is good to keep in mind,
quoting Bill Gates:
Software will be the single most important force in digital
entertainment over the next decade.
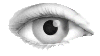
It should not come as a surprise that this statement is meant to promote a new
initiative, XNA,
which as the announcement says
is targeted to help contain the skyrocketing development costs and allow
developers to concentrate on the unique content that differentiates their games.
(C) Æliens
04/09/2009
You may not copy or print any of this material without explicit permission of the author or the publisher.
In case of other copyright issues, contact the author.